unsupervised_decomposition.hxx
|
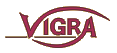 |
37 #ifndef VIGRA_UNSUPERVISED_DECOMPOSITION_HXX
38 #define VIGRA_UNSUPERVISED_DECOMPOSITION_HXX
41 #include "mathutil.hxx"
43 #include "singular_value_decomposition.hxx"
118 template <
class T,
class C1,
class C2,
class C3>
124 using namespace linalg;
126 int numFeatures =
rowCount(features);
129 vigra_precondition(numSamples >= numFeatures,
130 "principalComponents(): The number of samples has to be larger than the number of features.");
131 vigra_precondition(numFeatures >= numComponents && numComponents >= 1,
132 "principalComponents(): The number of features has to be larger or equal to the number of components in which the feature matrix is decomposed.");
133 vigra_precondition(
rowCount(fz) == numFeatures,
134 "principalComponents(): The output matrix fz has to be of dimension numFeatures*numComponents.");
136 "principalComponents(): The output matrix zv has to be of dimension numComponents*numSamples.");
138 Matrix<T> U(numSamples, numFeatures), S(numFeatures, 1), V(numFeatures, numFeatures);
141 for(
int k=0; k<numComponents; ++k)
164 : min_rel_gain(1e-4),
166 normalized_component_weights(true)
175 vigra_precondition(n >= 1,
176 "PLSAOptions::maximumNumberOfIterations(): number must be a positive integer.");
187 vigra_precondition(g >= 0.0,
188 "PLSAOptions::minimumRelativeGain(): number must be positive or zero.");
202 normalized_component_weights = v;
208 bool normalized_component_weights;
291 template <
class U,
class C1,
class C2,
class C3,
class Random>
293 pLSA(MultiArrayView<2, U, C1>
const & features,
294 MultiArrayView<2, U, C2> fz,
295 MultiArrayView<2, U, C3> zv,
296 Random
const& random,
297 PLSAOptions
const & options = PLSAOptions())
299 using namespace linalg;
301 int numFeatures =
rowCount(features);
304 vigra_precondition(numFeatures >= numComponents && numComponents >= 1,
305 "pLSA(): The number of features has to be larger or equal to the number of components in which the feature matrix is decomposed.");
306 vigra_precondition(
rowCount(fz) == numFeatures,
307 "pLSA(): The output matrix fz has to be of dimension numFeatures*numComponents.");
309 "pLSA(): The output matrix zv has to be of dimension numComponents*numSamples.");
312 UniformRandomFunctor<Random> randf(random);
319 double eps = 1.0/NumericTraits<U>::max();
320 double lastChange = NumericTraits<U>::max();
326 Matrix<U> columnSums(1, numSamples);
327 features.sum(columnSums);
328 Matrix<U> expandedSums = ones<U>(numFeatures, 1) * columnSums;
330 while(iteration < options.max_iterations && (lastChange > options.min_rel_gain))
332 Matrix<U> fzv = fz*zv;
340 Matrix<U> factor = features / pointWise(fzv + (U)eps);
341 zv *= (fz.transpose() * factor);
342 fz *= (factor * zv.transpose());
347 Matrix<U> model = expandedSums * pointWise(fzv);
351 lastChange =
abs((err-err_old) / (U)(err + eps));
359 if(!options.normalized_component_weights)
362 for(
int k=0; k<numSamples; ++k)
367 template <
class U,
class C1,
class C2,
class C3>
369 pLSA(MultiArrayView<2, U, C1>
const & features,
370 MultiArrayView<2, U, C2> & fz,
371 MultiArrayView<2, U, C3> & zv,
372 PLSAOptions
const & options = PLSAOptions())
374 RandomNumberGenerator<> generator(RandomSeed);
375 pLSA(features, fz, zv, generator, options);
383 #endif // VIGRA_UNSUPERVISED_DECOMPOSITION_HXX
PLSAOptions & minimumRelativeGain(double g)
Definition: unsupervised_decomposition.hxx:185
MultiArrayView< 2, T, C > columnVector(MultiArrayView< 2, T, C > const &m, MultiArrayIndex d)
Definition: matrix.hxx:727
MultiArrayIndex rowCount(const MultiArrayView< 2, T, C > &x)
Definition: matrix.hxx:671
Option object for the pLSA algorithm.
Definition: unsupervised_decomposition.hxx:158
PLSAOptions()
Definition: unsupervised_decomposition.hxx:163
void principalComponents(MultiArrayView< 2, T, C1 > const &features, MultiArrayView< 2, T, C2 > fz, MultiArrayView< 2, T, C3 > zv)
Decompose a matrix according to the PCA algorithm.
Definition: unsupervised_decomposition.hxx:120
void initMultiArray(...)
Write a value to every element in a multi-dimensional array.
MultiArrayView< N, T, StridedArrayTag > transpose() const
Definition: multi_array.hxx:1567
PLSAOptions & normalizedComponentWeights(bool v=true)
Definition: unsupervised_decomposition.hxx:200
doxygen_overloaded_function(template<...> void separableConvolveBlockwise) template< unsigned int N
Separated convolution on ChunkedArrays.
Matrix< T, ALLLOC >::SquaredNormType squaredNorm(const Matrix< T, ALLLOC > &a)
void pLSA(...)
Decompose a matrix according to the pLSA algorithm.
MultiArrayView< 2, T, C > rowVector(MultiArrayView< 2, T, C > const &m, MultiArrayIndex d)
Definition: matrix.hxx:697
void prepareColumns(...)
Standardize the columns of a matrix according to given DataPreparationGoals.
MultiArrayIndex columnCount(const MultiArrayView< 2, T, C > &x)
Definition: matrix.hxx:684
unsigned int singularValueDecomposition(MultiArrayView< 2, T, C1 > const &A, MultiArrayView< 2, T, C2 > &U, MultiArrayView< 2, T, C3 > &S, MultiArrayView< 2, T, C4 > &V)
Definition: singular_value_decomposition.hxx:75
linalg::TemporaryMatrix< T > abs(MultiArrayView< 2, T, C > const &v)
PLSAOptions & maximumNumberOfIterations(unsigned int n)
Definition: unsupervised_decomposition.hxx:173