rf_online_prediction_set.hxx
|
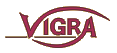 |
1 #include "../multi_array.hxx"
11 SampleRange(
int start,
int end,
int num_features)
15 this->min_boundaries.resize(num_features,-FLT_MAX);
16 this->max_boundaries.resize(num_features,FLT_MAX);
21 mutable std::vector<T> max_boundaries;
22 mutable std::vector<T> min_boundaries;
24 bool operator<(
const SampleRange& o)
const
31 class OnlinePredictionSet
35 OnlinePredictionSet(MultiArrayView<2,T,U>& features,
int num_sets)
37 this->features=features;
38 std::vector<int> init(features.shape(0));
39 for(
unsigned int i=0;i<init.size();++i)
41 indices.resize(num_sets,init);
42 std::set<SampleRange<T> > set_init;
43 set_init.insert(SampleRange<T>(0,init.size(),features.shape(1)));
44 ranges.resize(num_sets,set_init);
45 cumulativePredTime.resize(num_sets,0);
51 for(
unsigned int i=0;i<cumulativePredTime.size();++i)
53 if(cumulativePredTime[i]>cumulativePredTime[result])
61 void reset_tree(
int index)
63 index=index % ranges.size();
64 std::set<SampleRange<T> > set_init;
65 set_init.insert(SampleRange<T>(0,features.shape(0),features.shape(1)));
66 ranges[index]=set_init;
67 cumulativePredTime[index]=0;
70 std::vector<std::set<SampleRange<T> > > ranges;
71 std::vector<std::vector<int> > indices;
72 std::vector<int> cumulativePredTime;
73 MultiArray<2,T> features;
bool operator<(FixedPoint< IntBits1, FracBits1 > l, FixedPoint< IntBits2, FracBits2 > r)
less than
Definition: fixedpoint.hxx:512