projective_registration.hxx
|
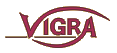 |
36 #ifndef VIGRA_PROJECTIVE_REGISTRATION_HXX
37 #define VIGRA_PROJECTIVE_REGISTRATION_HXX
39 #include "mathutil.hxx"
41 #include "linear_solve.hxx"
42 #include "tinyvector.hxx"
43 #include "splineimageview.hxx"
63 template <
class SrcPo
intIterator,
class DestPo
intIterator>
64 linalg::TemporaryMatrix<double>
73 vigra_assert(size >= 4,
74 "projectiveMatrix2DFromCorrespondingPoints(): need at least four corresponding points.");
76 vigra::Matrix<double> A(2*size,8, 0.0), b(2*size,1), res(8,1);
77 for (
int i =0; i<size; ++i, ++s, ++d)
81 A(i,0)=(*d)[0]; A(i,1)=(*d)[1]; A(i,2)=1; A(i,3)=0; A(i,4)=0; A(i,5)=0; A(i,6)=-1*((*d)[0])*((*s)[0]); A(i,7)=-1*((*d)[1])*((*s)[0]);
84 A(size+i,0)=0; A(size+i,1)=0; A(size+i,2)=0; A(size+i,3)=(*d)[0]; A(size+i,4)=(*d)[1]; A(size+i,5)=1; A(size+i,6)=-1*((*d)[0])*((*s)[1]); A(size+i,7)=-1*((*d)[1])*((*s)[1]);
91 static_cast<void>(solvable);
92 vigra_assert(solvable,
93 "projectiveMatrix2DFromCorrespondingPoints(): singular solution matrix.");
95 linalg::TemporaryMatrix<double> projectiveMat(3,3);
96 projectiveMat(0,0) = res(0,0); projectiveMat(0,1) = res(1,0); projectiveMat(0,2) = res(2,0);
97 projectiveMat(1,0) = res(3,0); projectiveMat(1,1) = res(4,0); projectiveMat(1,2) = res(5,0);
98 projectiveMat(2,0) = res(6,0); projectiveMat(2,1) = res(7,0); projectiveMat(2,2) = 1;
100 return projectiveMat;
191 template <
int ORDER,
class T,
192 class DestIterator,
class DestAccessor,
195 DestIterator dul, DestIterator dlr, DestAccessor dest,
196 MultiArrayView<2, double, C>
const & projectiveMatrix)
198 vigra_precondition(
rowCount(projectiveMatrix) == 3 &&
columnCount(projectiveMatrix) == 3 && projectiveMatrix(2,2) == 1.0,
199 "projectiveWarpImage(): matrix doesn't represent an projective transformation with homogeneous 2D coordinates.");
202 double w = dlr.x - dul.x;
203 double h = dlr.y - dul.y;
205 for(
double y = 0.0; y < h; ++y, ++dul.y)
207 typename DestIterator::row_iterator rd = dul.rowIterator();
208 for(
double x=0.0; x < w; ++x, ++rd)
210 double fac = 1.0/(x*projectiveMatrix(2,0) + y*projectiveMatrix(2,1) + 1);
211 double sx = (x*projectiveMatrix(0,0) + y*projectiveMatrix(0,1) + projectiveMatrix(0,2)) * fac;
212 double sy = (x*projectiveMatrix(1,0) + y*projectiveMatrix(1,1) + projectiveMatrix(1,2)) * fac;
213 if(src.isInside(sx, sy))
214 dest.set(src(sx, sy), rd);
219 template <
int ORDER,
class T,
220 class DestIterator,
class DestAccessor,
224 triple<DestIterator, DestIterator, DestAccessor> dest,
225 MultiArrayView<2, double, C>
const & projectiveMatrix)
231 template <
int ORDER,
class T,
236 MultiArrayView<2, T2, S2> dest,
237 MultiArrayView<2, double, C>
const & projectiveMatrix)
MultiArrayIndex rowCount(const MultiArrayView< 2, T, C > &x)
Definition: matrix.hxx:671
void projectiveWarpImage(...)
Warp an image according to an projective transformation.
linalg::TemporaryMatrix< double > projectiveMatrix2DFromCorrespondingPoints(SrcPointIterator s, SrcPointIterator send, DestPointIterator d)
Create homogeneous matrix that maps corresponding points onto each other.
Definition: projective_registration.hxx:65
doxygen_overloaded_function(template<...> void separableConvolveBlockwise) template< unsigned int N
Separated convolution on ChunkedArrays.
MultiArrayIndex columnCount(const MultiArrayView< 2, T, C > &x)
Definition: matrix.hxx:684