interpolating_accessor.hxx
|
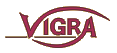 |
36 #ifndef VIGRA_INTERPOLATING_ACCESSOR_HXX
37 #define VIGRA_INTERPOLATING_ACCESSOR_HXX
40 #include "accessor.hxx"
80 template <
class ACCESSOR,
class VALUETYPE>
99 template <
class ITERATOR>
114 ret = a_(i,
Diff2D(ix, iy));
118 ret = detail::RequiresExplicitCast<value_type>::cast(
119 (1.0 - dy) * a_(i,
Diff2D(ix, iy)) +
120 dy * a_(i,
Diff2D(ix, iy + 1)));
127 ret = detail::RequiresExplicitCast<value_type>::cast(
128 (1.0 - dx) * a_(i,
Diff2D(ix, iy)) +
129 dx * a_(i,
Diff2D(ix + 1, iy)));
133 ret = detail::RequiresExplicitCast<value_type>::cast(
134 (1.0 - dx) * (1.0 - dy) * a_(i,
Diff2D(ix, iy)) +
135 dx * (1.0 - dy) * a_(i,
Diff2D(ix + 1, iy)) +
136 (1.0 - dx) * dy * a_(i,
Diff2D(ix, iy + 1)) +
137 dx * dy * a_(i,
Diff2D(ix + 1, iy + 1)));
148 template <
class ITERATOR>
155 return detail::RequiresExplicitCast<value_type>::cast(
156 (1.0 - dx) * (1.0 - dy) * a_(i,
Diff2D(ix, iy)) +
157 dx * (1.0 - dy) * a_(i,
Diff2D(ix + 1, iy)) +
158 (1.0 - dx) * dy * a_(i,
Diff2D(ix, iy + 1)) +
159 dx * dy * a_(i,
Diff2D(ix + 1, iy + 1)));
BilinearInterpolatingAccessor(ACCESSOR a)
Definition: interpolating_accessor.hxx:90
Two dimensional difference vector.
Definition: diff2d.hxx:185
Bilinear interpolation at non-integer positions.
Definition: interpolating_accessor.hxx:81
value_type unchecked(ITERATOR const &i, float x, float y) const
Definition: interpolating_accessor.hxx:149
value_type operator()(ITERATOR const &i, float x, float y) const
Definition: interpolating_accessor.hxx:100
VALUETYPE value_type
Definition: interpolating_accessor.hxx:86