graph_rag_project_back.hxx
|
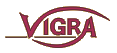 |
40 #ifndef VIGRA_GRAPH_RAG_PROJECT_BACK_HXX
41 #define VIGRA_GRAPH_RAG_PROJECT_BACK_HXX
52 #include "graph_generalization.hxx"
53 #include "multi_gridgraph.hxx"
54 #include "priority_queue.hxx"
55 #include "union_find.hxx"
56 #include "adjacency_list_graph.hxx"
57 #include "graph_maps.hxx"
64 namespace detail_rag_project_back{
68 class BASE_GRAPH_LABELS,
70 class BASE_GRAPH_FEATURES
72 struct RagProjectBack{
76 const AdjacencyListGraph & rag,
77 const BASE_GRAPH & bg,
78 const Int64 ignoreLabel,
79 const BASE_GRAPH_LABELS bgLabels,
80 const RAG_FEATURES & ragFeatures,
81 BASE_GRAPH_FEATURES & bgFeatures
83 typedef BASE_GRAPH Bg;
84 typedef typename Bg::NodeIt BgNodeIt;
85 typedef typename Bg::Node BgNode;
88 for(BgNodeIt iter(bg); iter!=lemon::INVALID; ++iter){
89 const BgNode bgNode(*iter);
90 bgFeatures[bgNode] = ragFeatures[rag.nodeFromId(bgLabels[bgNode])];
94 for(BgNodeIt iter(bg); iter!=lemon::INVALID; ++iter){
95 const BgNode bgNode(*iter);
96 if(static_cast<Int64>(bgLabels[bgNode])!=ignoreLabel)
97 bgFeatures[bgNode] = ragFeatures[rag.nodeFromId(bgLabels[bgNode])];
105 class BASE_GRAPH_LABELS,
107 class BASE_GRAPH_FEATURES
109 struct RagProjectBack<
110 vigra::GridGraph<3, undirected_tag>,
118 const AdjacencyListGraph & rag,
119 const BASE_GRAPH & bg,
120 const Int64 ignoreLabel,
121 const BASE_GRAPH_LABELS bgLabels,
122 const RAG_FEATURES & ragFeatures,
123 BASE_GRAPH_FEATURES & bgFeatures
125 typedef BASE_GRAPH Bg;
126 typedef typename Bg::Node BgNode;
135 for(
Int64 z=0; z<shape[2]; ++z){
138 for(node[1]=0; node[1]<shape[1]; ++node[1])
139 for(node[0]=0; node[0]<shape[0]; ++node[0]){
140 bgFeatures[node] = ragFeatures[rag.nodeFromId(bgLabels[node])];
147 for(
Int64 z=0; z<shape[2]; ++z){
150 for(node[1]=0; node[1]<shape[1]; ++node[1])
151 for(node[0]=0; node[0]<shape[0]; ++node[0]){
152 if(static_cast<Int64>(bgLabels[node])!=ignoreLabel)
153 bgFeatures[node] = ragFeatures[rag.nodeFromId(bgLabels[node])];
170 template<
class BASE_GRAPH,
171 class BASE_GRAPH_LABELS,
173 class BASE_GRAPH_FEATURES
177 const BASE_GRAPH & bg,
178 const Int64 ignoreLabel,
179 const BASE_GRAPH_LABELS bgLabels,
180 const RAG_FEATURES & ragFeatures,
181 BASE_GRAPH_FEATURES & bgFeatures
183 using namespace detail_rag_project_back;
184 detail_rag_project_back::RagProjectBack< BASE_GRAPH,BASE_GRAPH_LABELS,RAG_FEATURES,BASE_GRAPH_FEATURES>::projectBack(rag,
185 bg,ignoreLabel,bgLabels,ragFeatures,bgFeatures);
Define a grid graph in arbitrary dimensions.
Definition: multi_fwd.hxx:217
undirected adjacency list graph in the LEMON API
Definition: adjacency_list_graph.hxx:227
detail::SelectIntegerType< 64, detail::SignedIntTypes >::type Int64
64-bit signed int
Definition: sized_int.hxx:177
void projectBack(const AdjacencyListGraph &rag, const BASE_GRAPH &bg, const Int64 ignoreLabel, const BASE_GRAPH_LABELS bgLabels, const RAG_FEATURES &ragFeatures, BASE_GRAPH_FEATURES &bgFeatures)
Definition: graph_rag_project_back.hxx:175
Class for fixed size vectors.This class contains an array of size SIZE of the specified VALUETYPE...
Definition: accessor.hxx:940