gradient_energy_tensor.hxx
|
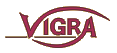 |
37 #ifndef VIGRA_GRADIENT_ENERGY_TENSOR_HXX
38 #define VIGRA_GRADIENT_ENERGY_TENSOR_HXX
43 #include "array_vector.hxx"
44 #include "basicimage.hxx"
45 #include "combineimages.hxx"
46 #include "numerictraits.hxx"
47 #include "convolution.hxx"
48 #include "multi_shape.hxx"
149 template <
class SrcIterator,
class SrcAccessor,
150 class DestIterator,
class DestAccessor>
152 DestIterator dupperleft, DestAccessor dest,
153 Kernel1D<double>
const & derivKernel, Kernel1D<double>
const & smoothKernel)
155 vigra_precondition(dest.size(dupperleft) == 3,
156 "gradientEnergyTensor(): output image must have 3 bands.");
158 int w = slowerright.x - supperleft.x;
159 int h = slowerright.y - supperleft.y;
162 NumericTraits<typename SrcAccessor::value_type>::RealPromote TmpType;
163 typedef BasicImage<TmpType> TmpImage;
164 TmpImage gx(w, h), gy(w, h),
165 gxx(w, h), gxy(w, h), gyy(w, h),
166 laplace(w, h), gx3(w, h), gy3(w, h);
168 convolveImage(srcIterRange(supperleft, slowerright, src), destImage(gx),
169 derivKernel, smoothKernel);
170 convolveImage(srcIterRange(supperleft, slowerright, src), destImage(gy),
171 smoothKernel, derivKernel);
173 derivKernel, smoothKernel);
175 smoothKernel, derivKernel);
177 smoothKernel, derivKernel);
179 std::plus<TmpType>());
181 derivKernel, smoothKernel);
183 smoothKernel, derivKernel);
184 typename TmpImage::iterator gxi = gx.begin(),
191 for(
int y = 0; y < h; ++y, ++dupperleft.y)
193 typename DestIterator::row_iterator d = dupperleft.rowIterator();
194 for(
int x = 0; x < w; ++x, ++d, ++gxi, ++gyi, ++gxxi, ++gxyi, ++gyyi, ++gx3i, ++gy3i)
196 dest.setComponent(
sq(*gxxi) +
sq(*gxyi) - *gxi * *gx3i, d, 0);
197 dest.setComponent(- *gxyi * (*gxxi + *gyyi) + 0.5 * (*gxi * *gy3i + *gyi * *gx3i), d, 1);
198 dest.setComponent(
sq(*gxyi) +
sq(*gyyi) - *gyi * *gy3i, d, 2);
203 template <
class SrcIterator,
class SrcAccessor,
204 class DestIterator,
class DestAccessor>
207 pair<DestIterator, DestAccessor> dest,
208 Kernel1D<double>
const & derivKernel, Kernel1D<double>
const & smoothKernel)
211 dest.first, dest.second, derivKernel, smoothKernel);
214 template <
class T1,
class S1,
218 MultiArrayView<2, T2, S2> dest,
219 Kernel1D<double>
const & derivKernel, Kernel1D<double>
const & smoothKernel)
221 vigra_precondition(src.shape() == dest.shape(),
222 "gradientEnergyTensor(): shape mismatch between input and output.");
224 destImage(dest), derivKernel, smoothKernel);
231 #endif // VIGRA_GRADIENT_ENERGY_TENSOR_HXX
void convolveImage(...)
Convolve an image with the given kernel(s).
void gradientEnergyTensor(...)
Calculate the gradient energy tensor for a scalar valued image.
NumericTraits< T >::Promote sq(T t)
The square function.
Definition: mathutil.hxx:382
void combineTwoImages(...)
Combine two source images into destination image.
doxygen_overloaded_function(template<...> void separableConvolveBlockwise) template< unsigned int N
Separated convolution on ChunkedArrays.