eccentricitytransform.hxx
|
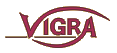 |
37 #ifndef VIGRA_ECCENTRICITYTRANSFORM_HXX
38 #define VIGRA_ECCENTRICITYTRANSFORM_HXX
45 #include "accumulator.hxx"
46 #include "multi_labeling.hxx"
47 #include "multi_distance.hxx"
48 #include "multi_resize.hxx"
49 #include "graph_algorithms.hxx"
56 template <
class Graph,
class WeightType,
57 class EdgeMap,
class Shape>
58 TinyVector<MultiArrayIndex, Shape::static_size>
59 eccentricityCentersOneRegionImpl(ShortestPathDijkstra<Graph, WeightType> & pathFinder,
60 const EdgeMap & weights, WeightType maxWeight,
61 Shape anchor, Shape
const & start, Shape
const & stop)
63 int maxIterations = 4;
64 for(
int k=0; k < maxIterations; ++k)
66 pathFinder.run(start, stop, weights, anchor, lemon::INVALID, maxWeight);
67 anchor = pathFinder.target();
71 Polygon<TinyVector<float, Shape::static_size> > path;
72 path.push_back_unsafe(anchor);
73 while(pathFinder.predecessors()[path.back()] != path.back())
74 path.push_back_unsafe(pathFinder.predecessors()[path.back()]);
75 return path[
roundi(path.arcLengthQuantile(0.5))];
78 template <
unsigned int N,
class T,
class S,
class Graph,
79 class ACCUMULATOR,
class DIJKSTRA,
class Array>
81 eccentricityCentersImpl(
const MultiArrayView<N, T, S> & src,
83 ACCUMULATOR
const & r,
84 DIJKSTRA & pathFinder,
88 typedef typename MultiArrayShape<N>::type Shape;
89 typedef typename Graph::Node Node;
90 typedef typename Graph::EdgeIt EdgeIt;
91 typedef float WeightType;
93 typename Graph::template EdgeMap<WeightType> weights(g);
94 WeightType maxWeight = 0.0,
97 AccumulatorChainArray<CoupledArrays<N, WeightType, T>,
98 Select< DataArg<1>, LabelArg<2>, Maximum> > a;
100 MultiArray<N, WeightType> distances(src.shape());
105 const Node u(g.u(*
edge)), v(g.v(*
edge));
106 const T label = src[u];
109 weights[*
edge] = NumericTraits<WeightType>::max();
113 WeightType weight =
norm(u - v) *
114 (get<Maximum>(a, label) + minWeight - 0.5*(distances[u] + distances[v]));
115 weights[*
edge] = weight;
116 maxWeight = std::max(weight, maxWeight);
120 maxWeight *= src.size();
122 T maxLabel = r.maxRegionLabel();
123 centers.resize(maxLabel+1);
125 for (T i=0; i <= maxLabel; ++i)
127 if(get<Count>(r, i) == 0)
129 centers[i] = eccentricityCentersOneRegionImpl(pathFinder, weights, maxWeight,
130 get<RegionAnchor>(r, i),
131 get<Coord<Minimum> >(r, i),
132 get<Coord<Maximum> >(r, i) + Shape(1));
172 template <
unsigned int N,
class T,
class S,
class Array>
179 typedef float WeightType;
184 AccumulatorChainArray<CoupledArrays<N, T>,
185 Select< DataArg<1>, LabelArg<1>,
189 eccentricityCentersImpl(src, g, a, pathFinder, centers);
234 template <
unsigned int N,
class T,
class S,
class Array>
243 typedef typename Graph::Node Node;
244 typedef typename Graph::EdgeIt EdgeIt;
245 typedef float WeightType;
247 vigra_precondition(src.
shape() == dest.
shape(),
248 "eccentricityTransformOnLabels(): Shape mismatch between src and dest.");
254 AccumulatorChainArray<CoupledArrays<N, T>,
255 Select< DataArg<1>, LabelArg<1>,
259 eccentricityCentersImpl(src, g, a, pathFinder, centers);
261 typename Graph::template EdgeMap<WeightType> weights(g);
264 const Node u(g.u(*
edge)), v(g.v(*
edge));
265 const T label = src[u];
267 weights[*
edge] = NumericTraits<WeightType>::max();
272 for (T i=0; i <= a.maxRegionLabel(); ++i)
273 if(get<Count>(a, i) > 0)
274 filtered_centers.push_back(centers[i]);
275 pathFinder.runMultiSource(weights, filtered_centers.begin(), filtered_centers.end());
276 dest = pathFinder.distances();
279 template <
unsigned int N,
class T,
class S>
282 MultiArrayView<N, S> dest)
284 ArrayVector<TinyVector<MultiArrayIndex, N> > centers;
293 #endif // VIGRA_ECCENTRICITYTRANSFORM_HXX
Define a grid graph in arbitrary dimensions.
Definition: multi_fwd.hxx:217
PowerSum< 0 > Count
Alias. Count.
Definition: accumulator-grammar.hxx:157
Int32 roundi(FixedPoint16< IntBits, OverflowHandling > v)
rounding to the nearest integer.
Definition: fixedpoint.hxx:1775
const difference_type & shape() const
Definition: multi_array.hxx:1648
Coord< FirstSeen > RegionAnchor
Alias. Anchor point (first point of the region seen by scan-order traversal.
Definition: accumulator-grammar.hxx:220
FFTWComplex< R >::NormType norm(const FFTWComplex< R > &a)
norm (= magnitude)
Definition: fftw3.hxx:1037
shortest path computer
Definition: graph_algorithms.hxx:297
Definition: array_vector.hxx:58
void extractFeatures(...)
Class for fixed size vectors.This class contains an array of size SIZE of the specified VALUETYPE...
Definition: accessor.hxx:940
std::pair< typename vigra::GridGraph< N, DirectedTag >::edge_descriptor, bool > edge(typename vigra::GridGraph< N, DirectedTag >::vertex_descriptor const &u, typename vigra::GridGraph< N, DirectedTag >::vertex_descriptor const &v, vigra::GridGraph< N, DirectedTag > const &g)
Return the pair (edge_descriptor, true) when an edge between vertices u and v exists, or (lemon::INVALID, false) otherwise (API: boost).
Definition: multi_gridgraph.hxx:2990
Coord< Range > BoundingBox
Alias. Rectangle enclosing the region, as a std::pair of coordinates.
Definition: accumulator-grammar.hxx:218
use direct and indirect neighbors
Definition: multi_fwd.hxx:188